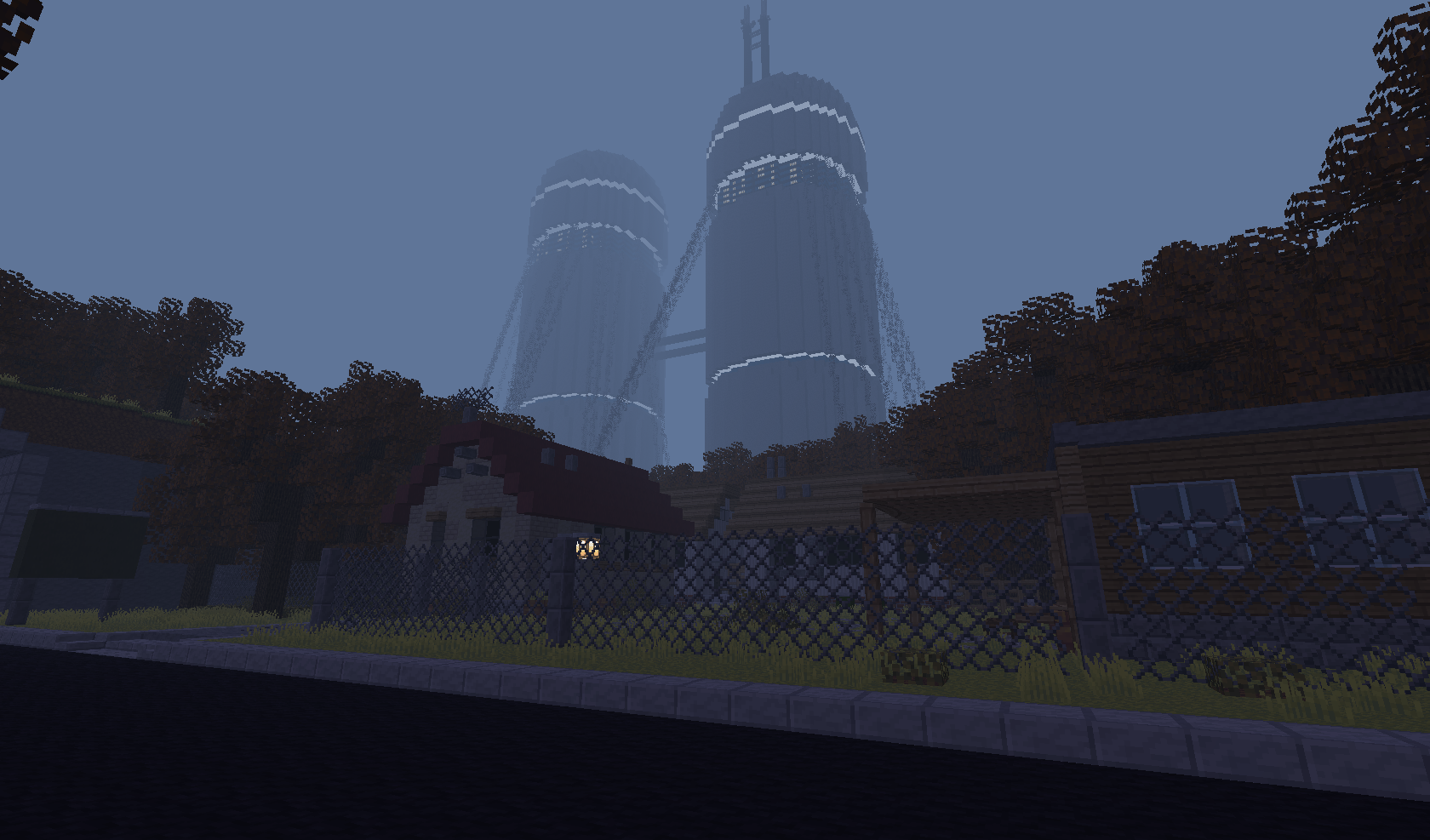
Everything posted by Goodly
-
Does MCGalaxy allow for saving in .mine/CW format?
What is the purpose of doing this?
-
Does MCGalaxy allow for saving in .mine/CW format?
No (it does not)
-
Modding the original server.jar
What's NMS?
-
Expanding the map of a server
Many basic building commands are explained here https://github.com/ClassiCube/MCGalaxy/wiki/Drawing
-
hello :)
You weren't banned for speaking spanish. You were banned because your post was only one word. No te banearon por hablar español. Te banearon porque tu publicación tenía solo una palabra.
-
how do you make custom blocks
You will need to use commands to add the block to the server after you have the textures you want to use. https://www.classicube.net/forum/viewpost/6048-custom_block_creation_a_user39s_field_guide/
-
Classicube Crashing
You should post any crash logs or crash message if there is any.
-
How do you make hd face skins
To add to this, make sure you resize by doubling only, such as 200% or 400% size
-
How to skin insert in mobile (Android)
Try visiting this url after you log in: https://www.classicube.net/acc
-
Can you ban someone?
They have either used /nickname or the server accepts any username and not just ClassiCube accounts
-
why does my skin show up as the default steve skin?
Can you please join AlwaysClassic so we can investigate this further? Solved, there were two issues: 1) AlwaysClassic is configured to use skins based on the Minecraft account that matches the ClassiCube account name. So if you don't own the same name on MC and CC it won't work like expected. 2) -own isn't valid syntax for resetting your skin to default
-
What is the nuke?
You can summon it using /hax summonentity tnt[tags:nuke]
- Now Accepting Plugin Requests
- Now Accepting Plugin Requests
-
Now Accepting Plugin Requests
MCGalaxy provides no way to accomplish point 1. As for a blacklist of maps that can't be teleported on rejoin, maybe any map with -hax shouldn't count as those are usually challenge maps with technical details that could get messed up
- Now Accepting Plugin Requests
-
Now Accepting Plugin Requests
Very nice, thank you! This actually showed me I can unregister default commands... I should do that instead of my hacky way to replace /highlight edit: shouldn't this be using p.ColoredName instead of p.DisplayName? edit2: Here is a version that fixes that: high5consent.cs
- Now Accepting Plugin Requests
-
Now Accepting Plugin Requests
Would you take requests for actual MCGalaxy PRs? I initially wanted a plugin to do this, but it really should be default behavior. A PR that: 1. allows the staff to choose which blocks are used in /highlight via a command (globally, only needs to be used once) 2. when highlighting is performed, replaces all existing highlight blocks in the level with another block 3. uses blockdefinitions, to lighten the chosen highlight blocks and darken all other fullbright blocks 3. temporarily darken the level using env cpe I wrote a plugin for NA2 that does everything except for step 1 if it helps. Though it currently assumes support for zone and blockdef and env CPE higherlight.cs
-
I am not accepting plugin requests
Out of boredom, I've decided, momentarily, that I will take a break from accepting plugin requests and start shitposting again. For perspective, I've created around 200+ shitposts over the years for both public and private forums and have done pretty much everything I can think of... which is where you guys come in. If you are looking for some shitposts for your forum or server or even just have a whacky/random idea that you think could be interesting, please leave a comment detailing your ideas below and I will evaluate whether or not it is worth attempting. Disclaimer 1: I will not be undertaking extremely time-consuming/high effort requests such as bee movie script, original copypastas, etc Disclaimer 2: I probably won't do this for very long depending on how many requests I get, but I will try my best. Disclaimer 3: I will not be creating shitposts different to those that already exist, either by myself or another user. Please check my post history to avoid unique requests. Disclaimer 4: Shitposts may take anywhere from a few days to a couple of weeks, depending on the difficulty of the shitpost and the amount of requests in the queue.
-
Does vandalizing counts as a not-do from the terms of use?
Grief and destroying levels is not typically considered a violation of the TOS. If you don't want your maps to be destroyed, I recommend that you avoid servers that have no rules (anarchy) such as the free owner one.
-
How to spawn mobs and models in CC?
To create bots read this page: https://github.com/ClassiCube/MCGalaxy/wiki/Bots To create the zombies made of blocks, use /physics 3, then /place zombie (or /zombie to toggle zombie-block placing mode) To see other special blocks, use /blocks complex all
-
minetest assets in classicube
What do you mean by self contained? ClassiCube already has its own set of original assets in situations where downloading the assets from Mojang isn't available. You're welcome to make your own texture pack based on minetest if you want though
-
I cannot connect to my old classic server via the classicube client
Turn Non-classic features OFF and then the Game version option should become available
-
I cannot connect to my old classic server via the classicube client